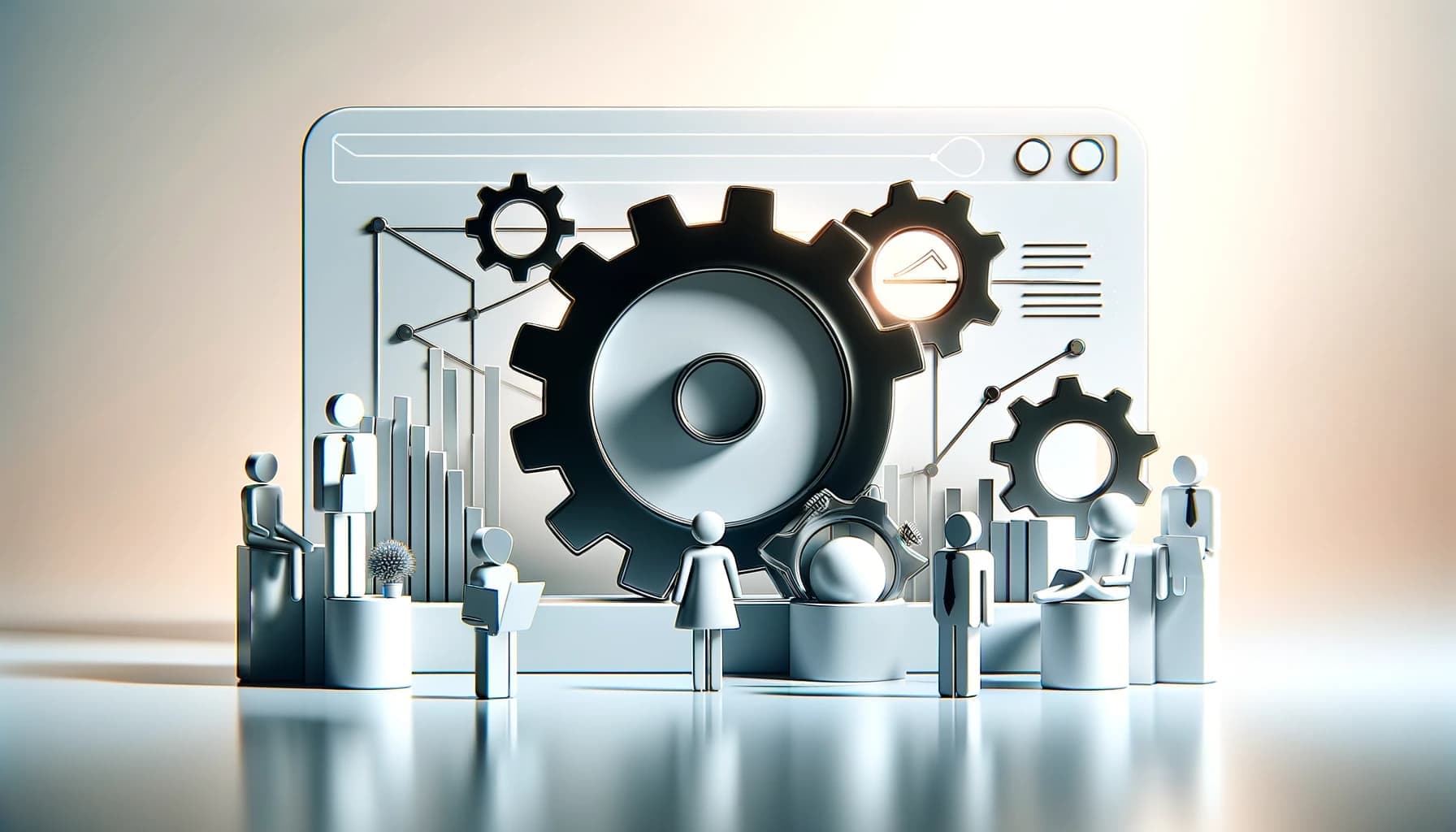
Strategic SEO for React Apps: Boosting Discoverability
Published on Dec 06, 2023Optimizing Search Engine Optimization (SEO) for a React app with client-side rendering poses unique challenges due to its Single Page App (SPA) nature, where React relies on just one HTML file. However, improving the SEO of your React app is not an insurmountable task. Here's my approach to boosting the SEO of my React app.
Create a Sitemap
Informing search engines about your site's structure is crucial. You can manually create a sitemap or utilize a package like react-router-sitemap. Here's an example of how to implement it:
// sitemap-routes.jsimport React from "react"import { BrowserRouter, Route } from "react-router-dom"export default (<BrowserRouter><Route path="/blogs" /><Route path="/blogs/:id" /><Route path="/about-us" /></BrowserRouter>)
And then create a function to map all your routes, dynamically handling parameters with react-router-sitemap:
// sitemap-builder.jsrequire("babel-register")({presets: ["es2015", "react"]})const router = require("./sitemap-routes").defaultconst Sitemap = require("react-router-sitemap").defaultasync function generateSitemap() {const blogs = await fetchBlogs()const blogsId = blogs.map(blog => ({ id: blog.id }))const paramsConfig = {'/blogs/:id': blogsId}return (new Sitemap(router).applyParams(paramsConfig).build("https://ibrahim.id").save("./public/sitemap.xml"))}
Utilize React Helmet
Enhance your React app's <head> tag using the react-helmet package. This package allows you to easily add title, base, meta, link, script, noscript, and style tags to your components. Example:
import React from "react"import { Helmet } from "react-helmet"function Application() {return (<div className="application"><Helmet><meta charSet="utf-8" /><title>My Title</title><link rel="canonical" href="http://ibrahim.id" /></Helmet>...</div>)}
Optimize Document Structure Tags
Structured content with proper heading tags hierarchy is preferred by search engine crawlers. Ensure a single H1 tag for each page to convey the content's main topic effectively.
Implement Schema Markup
Integrate Schema, a set of vocabularies readable by machines, to add meaning to your webpage's information. This can be done through various encodings, including RDFa, Microdata, and JSON-LD.
Remember, these steps form the basic foundation. To maximize results, consider actions such as submitting your site to search console, adding meta keywords, meta descriptions, and other SEO best practices.